Abstract: This applicaTIon note describes how to use a NonvolaTIle TImekeeping RAM with an 8051-type microcontroller. An example schemaTIc is provided. Source code provides an example of how to read and write the real-time clock (RTC) registers.
IntroductionThe nonvolatile timekeeping family of real-time clock (RTC) products provide battery-backed NV SRAM as well as time and date. The clock registers are accessed identically to the SRAM. Table 1 shows a list of products in the nonvolatile timekeeping family. The DS174_ series are identical to the DS164_, with the addition of a century bit and optional 3.3V operation.
Table 1. Nonvolatile Timekeeping Products
Part | RAM Density | VCC (V) |
DS1642 | 2k × 8 | 5 |
DS1643 | 8k × 8 | 5 |
DS1644 | 32k × 8 | 5 |
DS1646 | 128k × 8 | 5 |
DS1647 | 512k × 8 | 5 |
DS1742 | 2k × 8 | 3.3 or 5 |
DS1743 | 8k × 8 | 3.3 or 5 |
DS1744 | 32k × 8 | 3.3 or 5 |
DS1746 | 128k × 8 | 3.3 or 5 |
DS1747 | 512k × 8 | 3.3 or 5 |
Pin Configurations
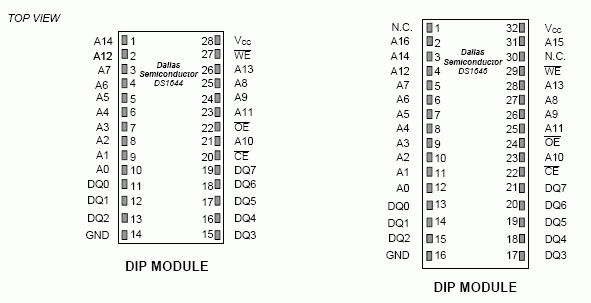
In the following example, a DS1644 or DS1646 is connected to a DS87C320. The DS87C320 high-speed microcontroller is compatible with the industry-standard 8051 architecture. Other NV RAM densities could be supported with appropriate changes to the circuit. For related application notes, please refer to the Quick View data sheets for the DS87C320 and DS1644. A reference RTC clock shematic follows the code.
DS1644 / DS1646 Code / ********************************************* ************************* / / * DS1644.c-Access DS1644 / 6, DS1744 / 6 using Reference RTC circuit * / / * This program if for example only and is not supported by Dallas * / / * Semiconductor Maxim. * / / ****************************** **************************************** / #include
/ * Prototypes for I / O functions * / #include / * Register declarations for DS5000 * / #include / * needed to define xdata addresses * / #include / ************************* bit definitions ********************** ****** / / ***************************** Defines ************ ******************** / / ************************* Global Variables * ************************** / uchar yr, mn, dt, dy, hr, min, sec, day; unsigned int MAXADD; sbit RSTb = P2 ^ 7; sbit IRQb = P2 ^ 6; sbit AD16 = P1 ^ 5; sbit AD17 = P1 ^ 6; sbit AD18 = P1 ^ 7; / **************** ******* Function Prototypes ************************** / void writereg (); void init_rtc (); void Disp_Clk_Regs () ; void ramread (); void ramwrite (); void toggle_ft (); void init_rtc () / * ------------ set the time and date ----------- ------ * / / * Note: NO error checking is done on the user entries! * / {Uchar tmp; printf ("Enter the year (0-99):"); scanf ("% bx", & yr); printf ("Enter the month (1-12):"); scanf ("% bx", & mn); printf ("Enter the date (1-31):"); scanf ("% bx", & dt); printf ("Enter the day (1-7):"); scanf ("% bx", & dy); printf ("Enter the hour (1-23):"); scanf ("% bx", & hr); printf ("Enter the minute (0-59): "); scanf ("% bx ", & min); printf (" Enter the second (0-59): "); scanf ("% bx ", & sec); AD16 = AD17 = AD18 = 1; XBYTE [0xfff8] = 0x80; / * set write bit for write * / XBYTE [0xfff9] = sec; XBYTE [0xfffa] = min; XBYTE [0xfffb] = hr; XBYTE [0xfffc] = dy; XBYTE [0xfffd] = dt; XBYTE [0xfffe ] = mn; XBYTE [0xffff] = yr; XBYTE [0xfff8] = 0; / * clear write bit to allow update * /} void Disp_Clk_Regs () / * --------------- -------------------------- * / {uchar msec, Sec, prv_sec = 99, Min, Hrs, Dte, Mon, Day, Yr; AD16 = AD17 = AD18 = 1; printf ("Yr Mn Dt Dy Hr: Mn: Sec"); while (! RI) / * Read & Display Clock Registers * / {XBYTE [0xfff8] = 0x40; / * set read bit to stop updates * / Sec = XBYTE [0xfff9]; Min = XBYTE [0xfffa]; Hrs = XBYTE [0xfffb]; Day = XBYTE [0xfffc]; Dte = XBYTE [0xfffd]; Mon = XBYTE [0xfffe]; Yr = XBYTE [0xffff]; XBYTE [0xfff8] = 0; / * clear read bit to resume updates * / delay (3); / * must allow time for transfer to occur * / if (Sec! = Prv_sec) / * display every time seconds change * / {printf ("% 02.bX% 02.bX% 02.bX % 02.bX ", Yr, Mon, Dte, Day); printf ("% 02.bX:% 02.bX:% 02.bX ", Hrs, Min, Sec);} prv_sec = Sec;} RI = 0 ; / * Swallow keypress to exit loop * /} void ramread () / * ------------ Read RAM ------------- * / {unsigned int j ; AD16 = AD17 = AD18 = 0; for (j = 0; j <= MAXADD; j ++) {if (j! = 0 &&! (J% 256)) {printf ("Press a key or Esc to exit") ; if (_getkey () == 0x1b) return;} if (! (j% 16)) printf ("% bx% 04x", (uchar) AD16, j); printf ("% 02.bx", (uchar ) XBYTE [j]); if (j == 0xffff) break;} if (MAXADD == 0xffff) {printf ("Press a key or Esc to exit"); if (_getkey () == 0x1b) return; AD16 = 1; for (j = 0; j <= MAXADD; j ++) if (j! = 0 &&! (J% 256)) {printf ("Press a key or Esc to exit"); if (_getkey () = = 0x1b) break;} if (! (J% 16)) printf ("% bx% 04x", (uchar) AD16, j); printf ("% 02.bx", (uchar) XBYTE [j]); if (j == 0xffff) break;}}} void ramwrite () / * ------------- write with incrementing data ------------- * / {unsigned int j; uchar offset = 0; if (MAXADD == 0xFFFF) {AD16 = AD17 = AD18 = 0; for (j = 0; j <= MAXADD; j ++) {XBYTE [j] = (uchar) ((j & 0xff) + offset); if ((j & 0xff) == 0xff) offset ++; if (j == 0xffff) break;}} AD16 = 1; offset = 0; for (j = 0; j <(MAXADD-7); j ++) {XBYTE [j] = (uchar) ((j & 0xff) + offset); if ((j & 0xff) == 0xff) offset ++;}} void ramfill (uchar dat) / * ------------- fill with fixed data ----------- -* / {unsigned int j; if (MAXADD == 0xFFFF) {AD16 = AD17 = AD18 = 0; for (j = 0; j <= MAXADD; j ++) {if (j & 0x100) XBYTE [j] = dat ^ 0xFF; else XBYTE [j] = dat; if (j == 0xffff) break;}} AD16 = 1; for (j = 0; j <(MAXADD-7); j ++) / * don't disturb time & date * / {if (j & 0x100) XBYTE [j] = dat ^ 0xFF; else XBYTE [j] = dat;}} void toggle_ft () / * -------- toggle the Frequency Test bit- -------- * / {uchar creg; AD16 = 1; creg = XBYTE [0xfffc]; if ((creg & 0x40)) / * check FT bit * / {XBYTE [0xfff8] = 0x80; / * set write bit for write * / XBYTE [0xfffc] = (creg & 0xbf); / * clear FT bit * / XBYTE [0xfff8] = 0; / * clear write bit * /} else {XBYTE [0xfff8] = 0x80; / * set write bit for write * / XBYTE [0xfffc] = (creg | 0x40); / * set FT bit * / XBYTE [0xfff8] = 0; / * clear write bit * /}} main (void) / * ------------------------------------------------ ----- * / {uchar i, M, M1; RSTb = 1; printf ("1) DS1644 or 2) DS1646?"); i = _getkey (); if (i == '1') MAXADD = 0x7FFF; if (i == '2') MAXADD = 0xFFFF; while (1) {printf ("DS1644 / 6 build 100"); printf ("CI Init clock CR Read Time"); printf ("R RAM Fill RR RAM Read "); printf (" T Toggle FT "); printf (" Enter Menu Selection: "); M = _getkey (); switch (M) {case 'C': case 'c': printf (" Enter Clock Routine to run: C "); M1 = _getkey (); switch (M1) {case 'I': case 'i': init_rtc (); break; case 'R': case 'r': Disp_Clk_Regs (); break ;} break; case 'R': case 'r': printf ("Fill RAM A) a, 5) 5, I) nc data or R) ead:"); M1 = _getkey (); switch (M1) { case '5': ramfill (0x55); break; case 'A': case 'a': ramfill (0xaa); break; case 'R': case 'r': ramread (); break; case 'I': case 'i': ramwrite (); break;} break; case 'T': case 't': toggle_ft (); break;}}} 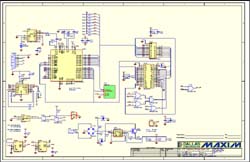
More Detailed Image
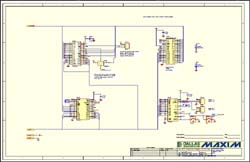
More Detailed Image
When the semiconductor diode is turned on, it is equivalent to the switch closing (circuit is turned on), and when it is turned off, it is equivalent to the switch opening (circuit cut), so the diode can be used for switching, and the commonly used model is 1N4148. Since the semiconductor diode has a unidirectional conduction characteristic, the PN junction is turned on under a positive bias, and the resistance in the on state is small, about several tens to several hundreds of ohms; in the reverse bias, it is off. The resistance is very large. Generally, the silicon diode is above 10 Μ Ω, and the bismuth tube is also several tens of kilo ohms to several hundred kilo ohms. With this feature, the diode will act as a control for the current to turn on or off in the circuit, making it an ideal electronic switch.
Switching Diode
Switching Diode,1N4148 Signal Diode,4148 Diode,1N4148 Diode
Dongguan Agertech Technology Co., Ltd. , https://www.agertechcomponents.com